Getting started
Step 1: Setup the development environment​
What you need before starting​
- Your AWS account is SSO-enabled to access https://deltatre.awsapps.com/start. After being SSO-enabled (please refer to your Project Manager), restart your development machine.
Setup​
- Access https://deltatre.awsapps.com/start and select
Access keys
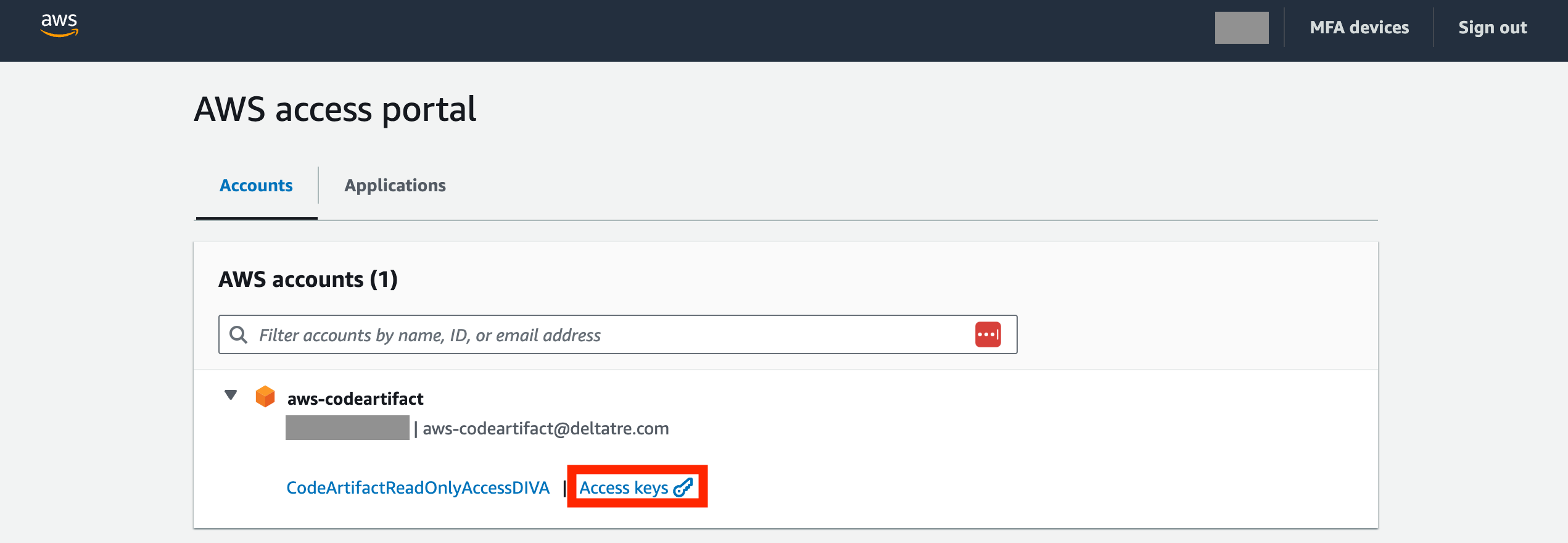
-
In a terminal, execute the three imports in
Option 1: Set AWS environment variables
-
Go back to https://deltatre.awsapps.com/start and select
CodeArtifactReadOnlyAccessDIVA
-
Search and select
CodeArtifact
-
Select the
Diva
repository, filter byFormat:maven
, and selectView connection instructions
-
Select
Operating system
,Gradle
as the package manager, SelectPull from your repository
as configuration method, and copy the login command in Step 3
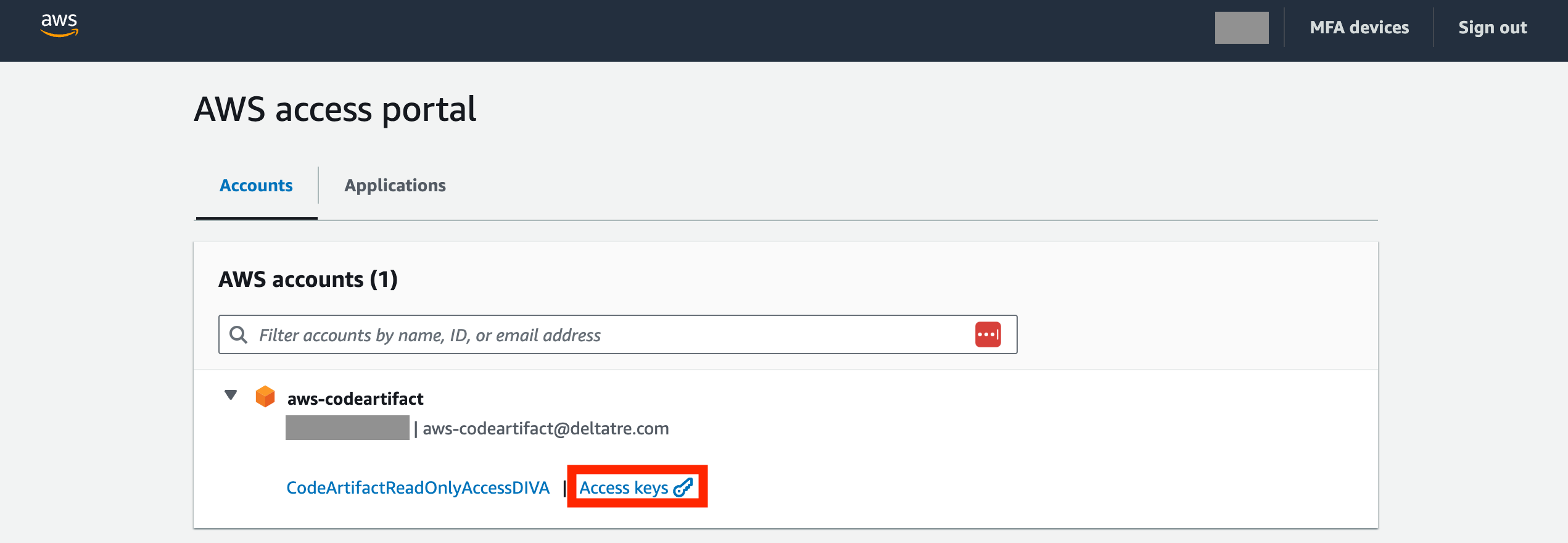
-
Generate the authorisation token running the copied command, like in the following example:
export CODEARTIFACT_AUTH_TOKEN=`aws codeartifact get-authorization-token --domain deltatre-diva --domain-owner <YOUR USER CODE> --region eu-central-1 --query authorizationToken --output text`
Note: The Authorisation Token expires within 12 hours. Repeat the steps until the login command every time you need to update the DIVA package.
- Add this Maven section to the dependencyResolutionManagement -> repositories section in the project's
settings.gradle
file
maven
{
url 'https://deltatre-diva-058264107880.d.codeartifact.eu-central-1.amazonaws.com/maven/Diva/'
credentials {
username "aws"
password System.env.CODEARTIFACT_AUTH_TOKEN
}
}
Step 2: Write your very first Android app with DIVA Player​
What you need before starting​
- Ensure the DIVA Back Office instance you rely on is up and running.
- Ask your video engineers team the
<video id>
and<settings URL>
. - In the
settings.gradle
file, in the repositories section, add:maven { url 'https://jitpack.io' }
maven { url "https://npaw.jfrog.io/artifactory/youbora/" }
Basic instantiation code (Mobile)​
In the build.gradle (Module :app)
file, add the following dependency:
implementation 'com.deltatre.diva:diva-mobile-divaboadapter:<latest-version>'
Under the layout
folder, in the activity_main.xml
file, add a fragment container view to the layout file:
<androidx.fragment.app.FragmentContainerView
android:id="@+id/fragment_container_view"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
In the AndroidManifest.xml
file, add the PiP capability to the Activity that contains the DIVA fragment (because DIVA requires the Picture-in-Picture feature):
<activity
...
android:supportsPictureInPicture="true">
...
</activity>
Given <videoId>
, <settingsURL>
, <languageCountryCode>
, <userToken>
and <sharedKey>
the following code (MainActivity.kt) streams the corresponding video:
package com.example.divaplayerhelloworld20
import android.os.Bundle
import androidx.activity.enableEdgeToEdge
import androidx.appcompat.app.AppCompatActivity
import androidx.core.view.ViewCompat
import androidx.core.view.WindowInsetsCompat
import com.deltatre.divaboadapter.DivaBoAdapterMobile
import com.deltatre.divaboadapter.DivaExtraParamsMobile
import androidx.lifecycle.lifecycleScope
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge()
setContentView(R.layout.activity_main)
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main)) { v, insets ->
val systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars())
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom)
insets
}
val divaExtraParameters = DivaExtraParamsMobile()
val boAdapter = DivaBoAdapterMobile(this /*refers to the Activity class */, lifecycleScope)
boAdapter.createDivaFragment(
adapterSettingsUrl = "<settingsURL>",
langCountryCode = "<languageCountryCode>",
videoId = "<videoId>",
getCurrentUserAccountAccessToken = {
//Get the user token
"<userToken>"
},
sharedKey = "<sharedKey>",
conf = divaExtraParameters,
version = null,
onError = { exception ->
//On Error
}) { divaFragment ->
supportFragmentManager
.beginTransaction()
.replace(R.id.fragment_container_view, divaFragment)
.commit()
}
}
}
Basic instantiation code (TV)​
In the build.gradle (Module :app)
file, add the following dependency:
implementation 'com.deltatre.diva:diva-tv-divaboadapter:<latest-version>'
Under the layout
folder, in the activity_main.xml
file, add a fragment container view to the layout file:
<androidx.fragment.app.FragmentContainerView
android:id="@+id/fragment_container_view"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
In the AndroidManifest.xml
file, add the LeanBack support as following:
<uses-feature
android:name="android.software.leanback"
android:required="true" />
<activity
...
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LEANBACK_LAUNCHER" />
</intent-filter>
...
</activity>
Given <videoId>
, <settingsURL>
, <languageCountryCode>
, <userToken>
and <sharedKey>
the following code (MainActivity.kt) streams the corresponding video:
package com.example.divaplayerhelloworld20
import android.os.Bundle
import androidx.activity.enableEdgeToEdge
import androidx.appcompat.app.AppCompatActivity
import androidx.core.view.ViewCompat
import androidx.core.view.WindowInsetsCompat
import com.deltatre.divaboadapter.DivaBoAdapterTV
import com.deltatre.divaboadapter.DivaExtraParams
import androidx.lifecycle.lifecycleScope
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val divaExtraParameters = DivaExtraParams()
val boAdapter = DivaBoAdapterTV(this /*refers to the Activity class */, lifecycleScope)
boAdapter.createDivaFragment(
adapterSettingsUrl = "<settingsURL>",
langCountryCode = "<languageCountryCode>",
videoId = "<videoId>",
getCurrentUserAccountAccessToken = {
//Get the user token
"<userToken>"
},
sharedKey = "<sharedKey>",
conf = divaExtraParameters,
version = null
onError = { exception ->
//On Error
}) { divaFragment ->
supportFragmentManager
.beginTransaction()
.replace(R.id.fragment_container_view, divaFragment)
.commit()
}
}
}