Getting started
Step 1: Setup the development environment​
What you need before starting​
- Your AWS account is SSO-enabled to access https://deltatre.awsapps.com/start. After being SSO-enabled (please refer to your Project Manager), restart your development machine.
Setup​
- Access https://deltatre.awsapps.com/start and select
Access keys
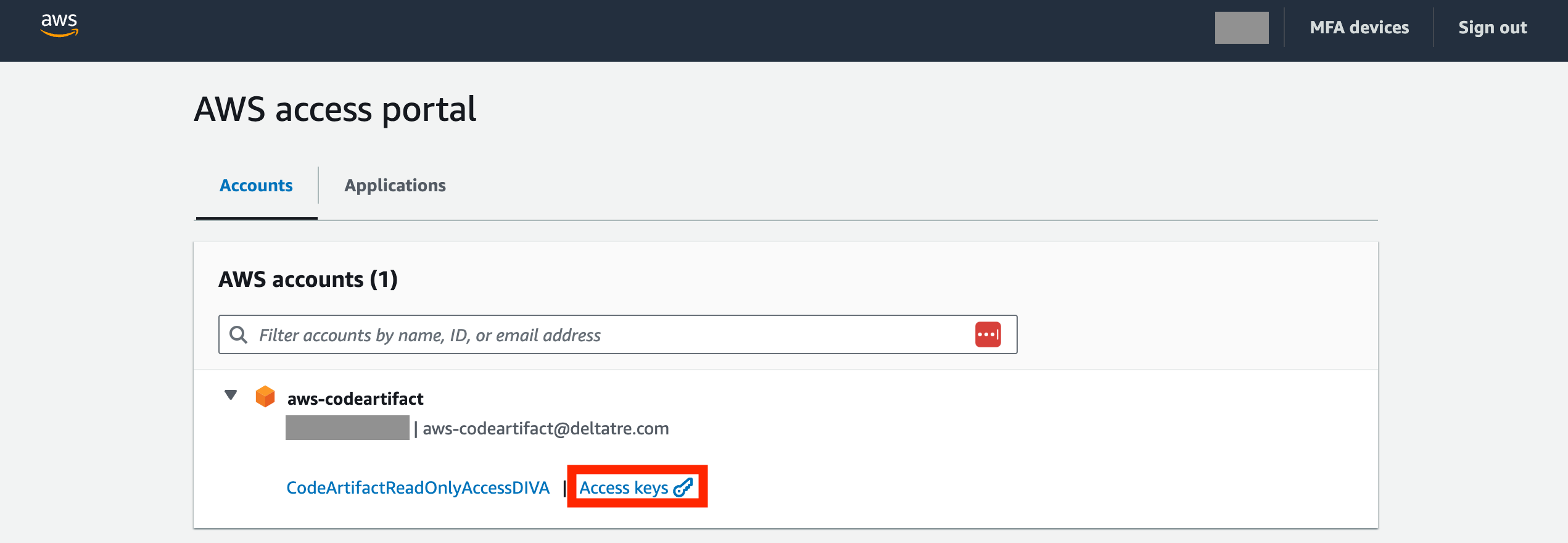
- In a terminal, execute the three imports in
Option 1: Set AWS environment variables
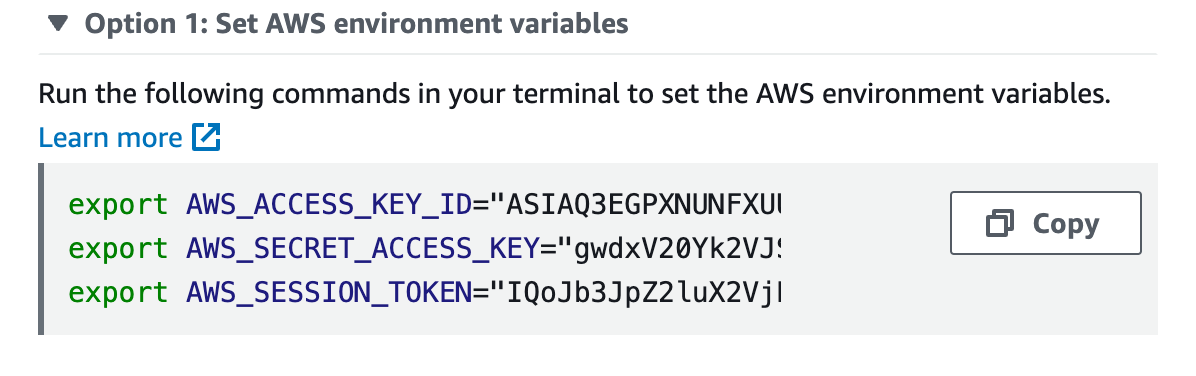
- Go back to https://deltatre.awsapps.com/start and select
CodeArtifactReadOnlyAccessDIVA
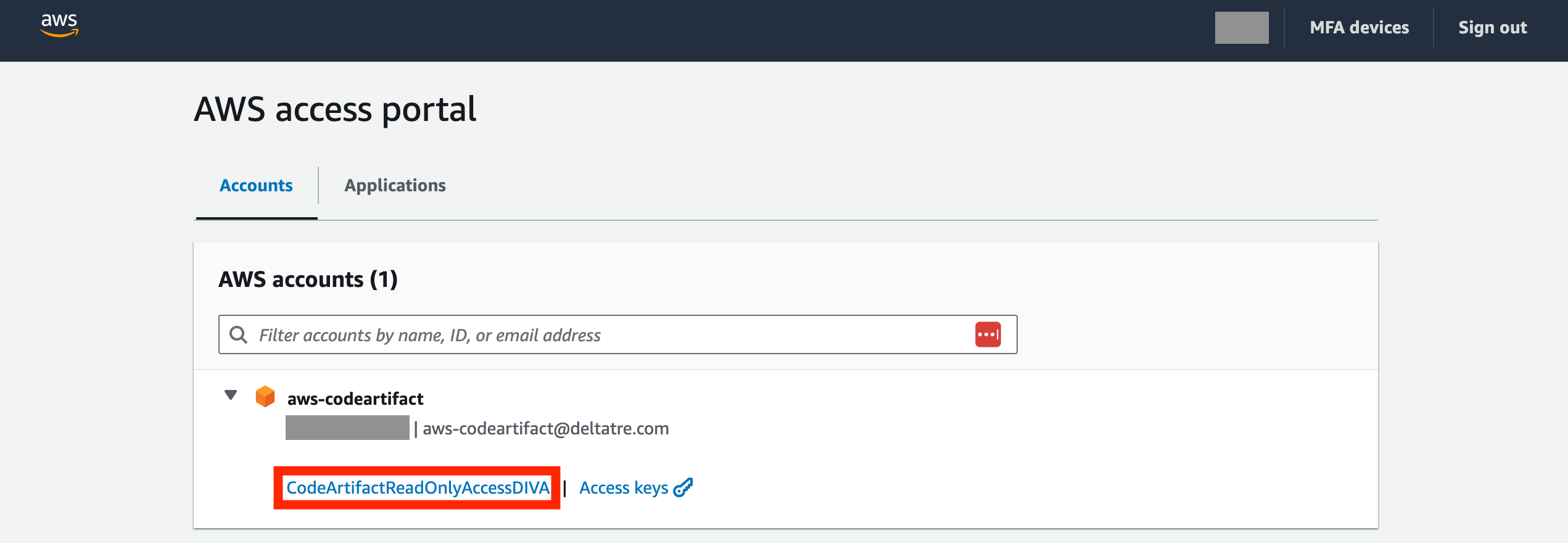
- Search and select
CodeArtifact
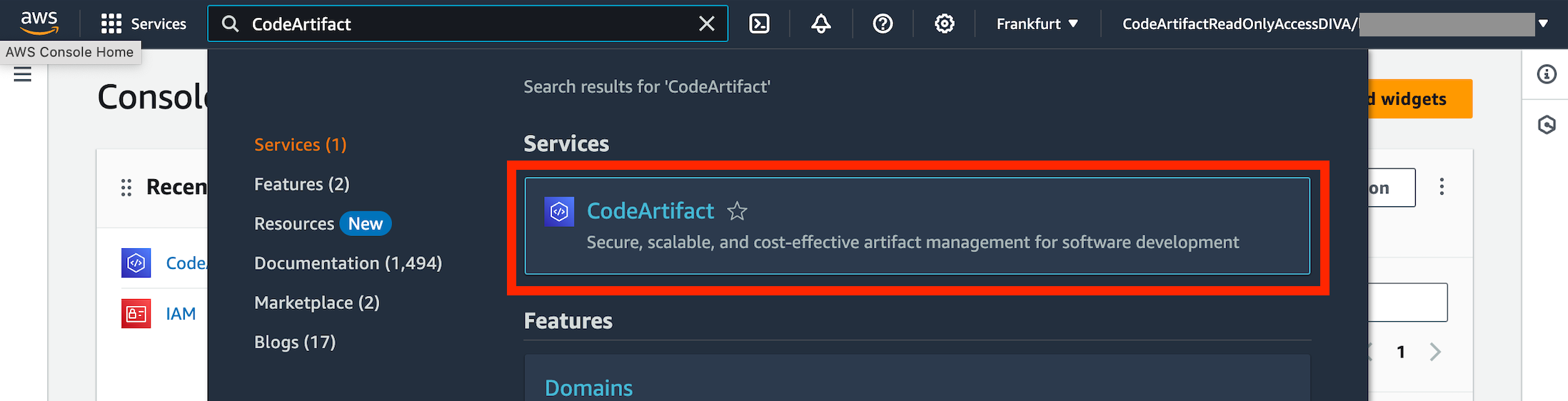
- Select the
Diva
repository, filter byFormat:npm
, and selectView connection instructions
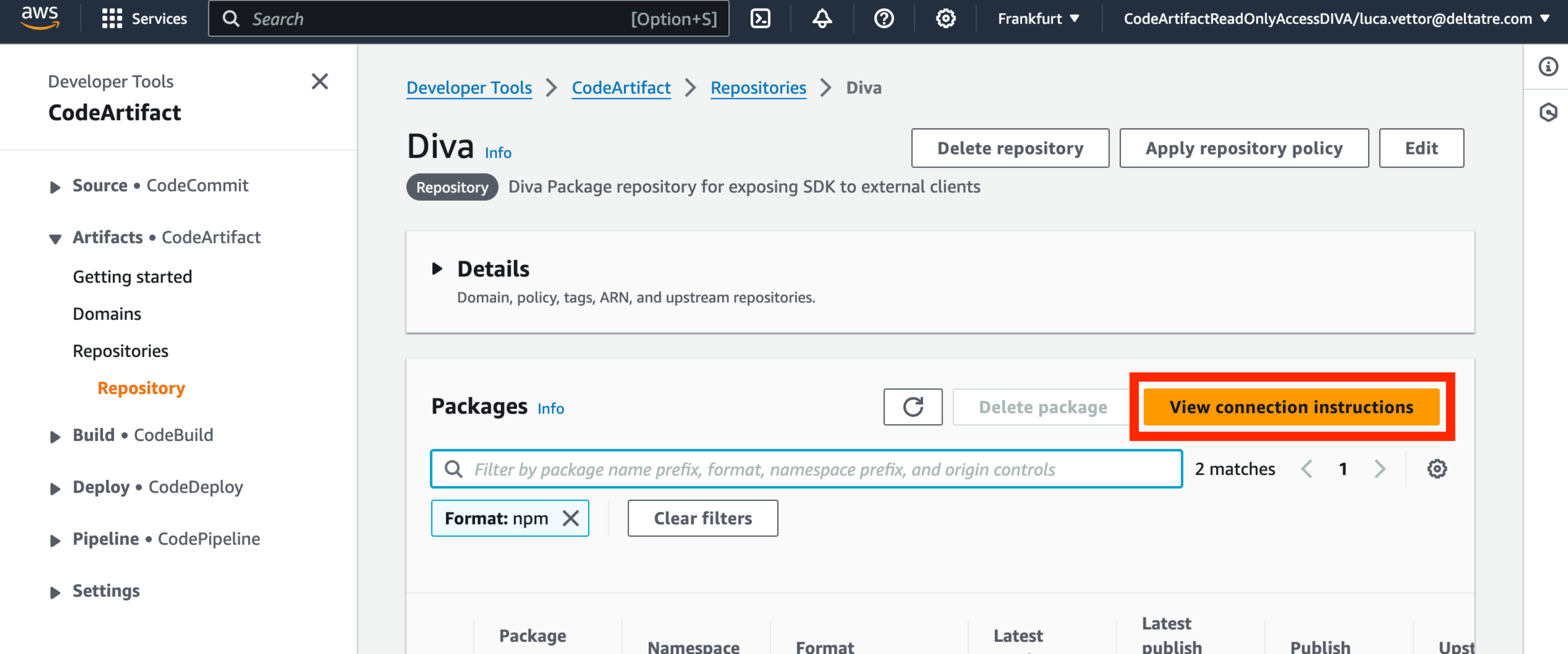
- Select
Operating system
,npm
as the package manager,Recommended method: configure using AWS CLI
, and copy the login command in Step 3
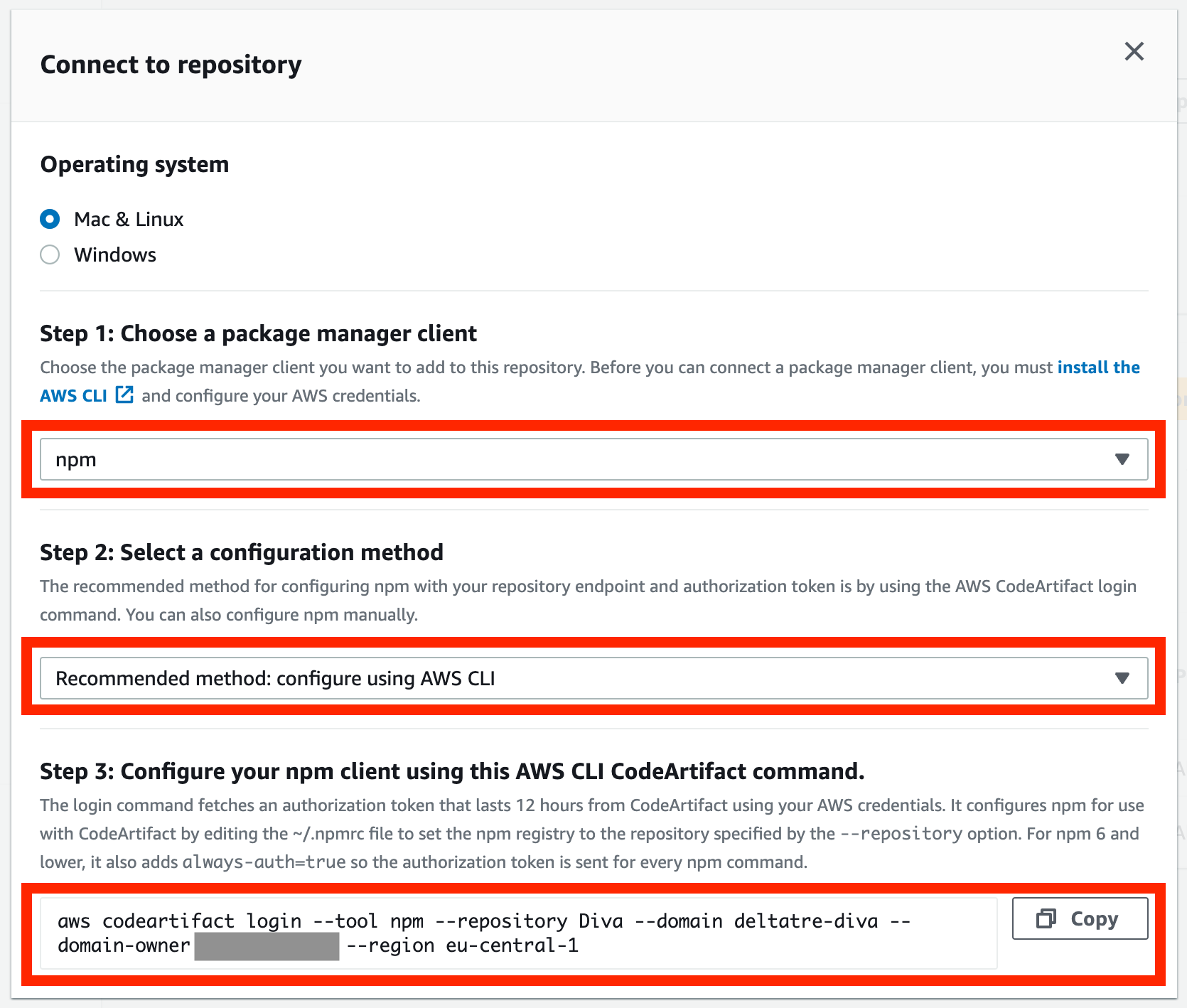
-
Before executing the login command, append
--namespace @deltatre-vxp
at the end, likeaws codeartifact login --tool npm --repository Diva --domain deltatre-diva --domain-owner <YOUR USER CODE> --region eu-central-1 --namespace @deltatre-vxp
Note: The login session expires within 12 hours. Repeat the steps until the login command every time you need to update the DIVA package.
-
Select your project folder and run command
npm i @deltatre-vxp/diva-sdk
-
You're ready to get started coding the front-end with the DIVA Player SDK.
Notes​
In your front-end js/typescript app, make sure to remove the tag <React.StrictMode>
from the root.render
function in the index.tsx
file. Otherwise, the video player's sound may be out of control.
Step 2: Write your very first web app with DIVA Player​
What you need before starting​
- Ensure the DIVA Back Office instance you rely on is up and running.
- Ask your video engineers team the
<video id>
and<settings URL>
.
Instantiation​
Replace <video id>
and <settings URL>
with values you got from your video engineers team in the following code:
import { DivaWebBoAdapter } from "@deltatre-vxp/diva-sdk/diva-web-bo-adapter";
import "@deltatre-vxp/diva-sdk/diva-web-sdk/index.min.css";
const videoId = "<video id>";
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.8.5/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: false,
googleDAI: false,
googleCast: false,
threeJs: false
};
const config = {
videoId: videoId,
libs: libs,
};
const props = {
settingsUrl: "<settings URL>",
languageCode: "en-US",
languageDictionary: "en-US",
onDivaBoAdapterError: console.error,
config: config
}
function App() {
return (
<DivaWebBoAdapter
{
...props
}
/>
);
}
export default App;