Getting started
Step 1: Setup the development environment​
What you need before starting​
- Your AWS account is SSO-enabled to access https://deltatre.awsapps.com/start. After being SSO-enabled (please refer to your Project Manager), restart your development machine.
Setup​
- Access https://deltatre.awsapps.com/start and select
Access keys
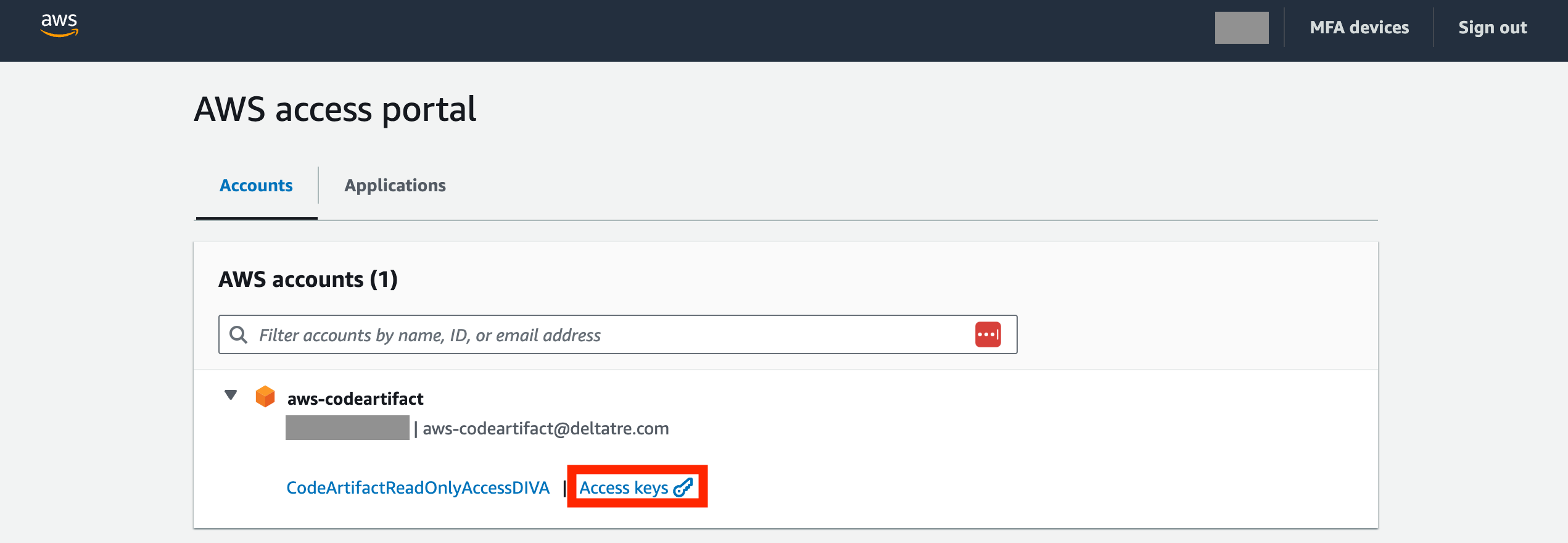
- In a terminal, execute the three imports in
Option 1: Set AWS environment variables
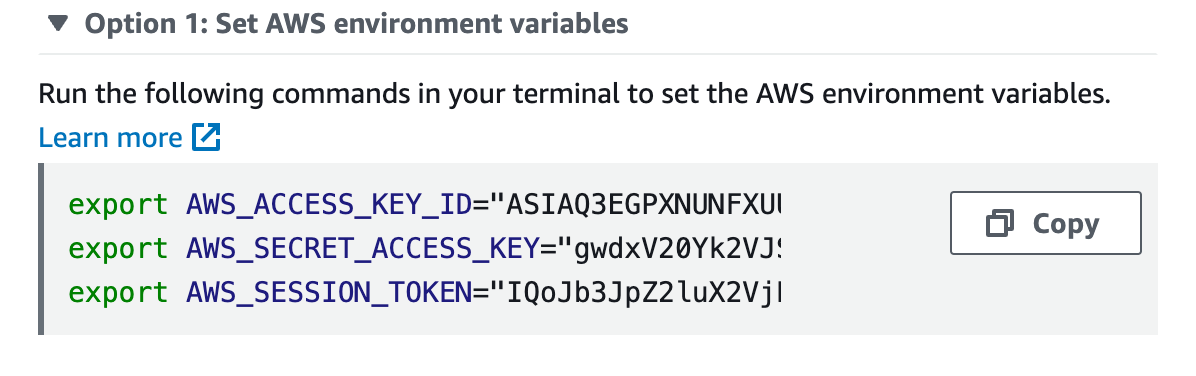
- Go back to https://deltatre.awsapps.com/start and select
CodeArtifactReadOnlyAccessDIVA
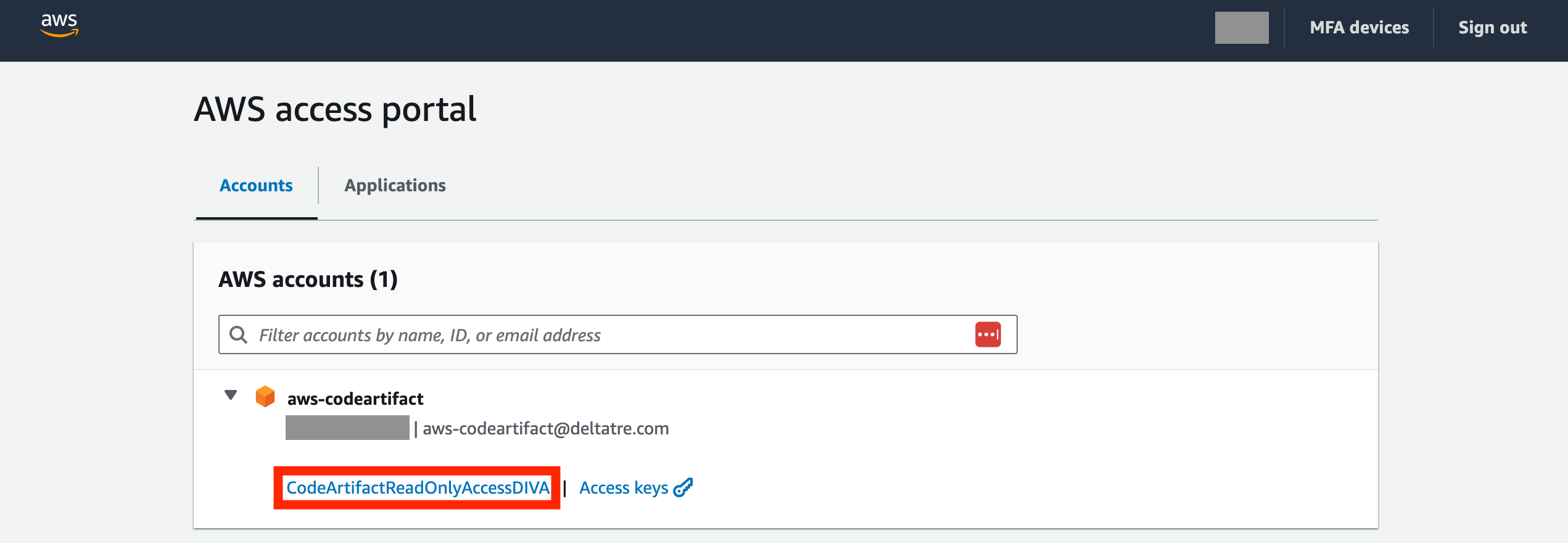
- Search and select
CodeArtifact
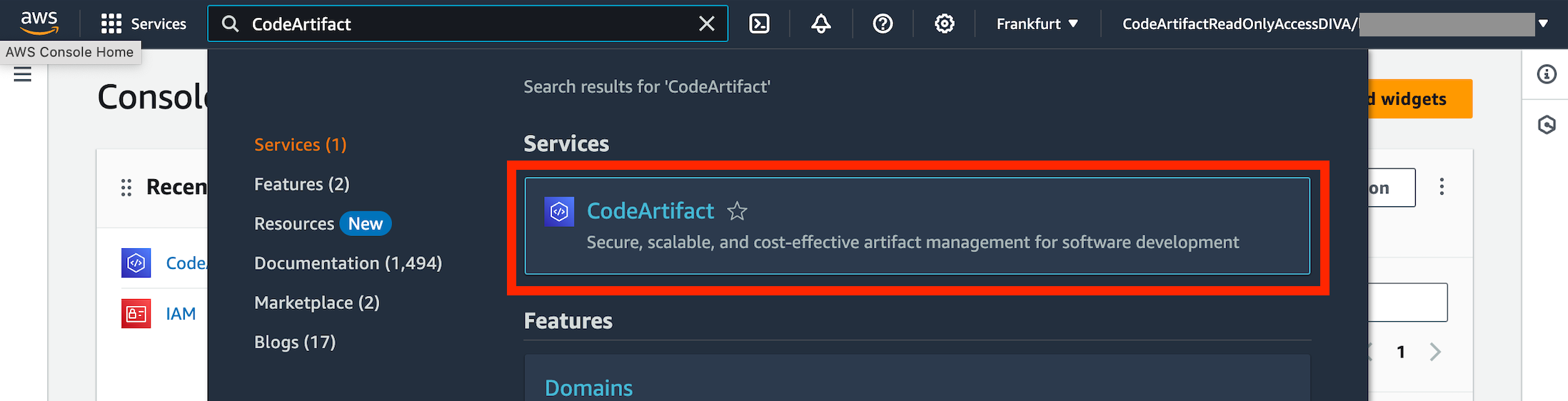
- Download and Install
AWS CLI
: Follow the instructions on the AWS CLI installation page for your operating system. The AWS CLI v2 is recommended.
Once installed, you can verify the installation by running:
aws --version
- Run AWS Configure: Open your terminal and run the following command:
aws configure
-
Enter your AWS_ACCESS_KEY_ID, AWS_SECRET_ACCESS_KEY, Default region name, and Default output format as prompted.
-
Configure your swift client using this AWS CLI CodeArtifact command. The login command fetches an authorization token from CodeArtifact using your AWS credentials and lasts 12 hours. It configures swift for use with CodeArtifact by editing ~/.swiftpm/configuration/registries.json and updating credentials. inside of the json you should see
diva
registry. This tells SPM to search for the dependencies using AWS package registry services. In order to do this you will need an existing package downloaded in your local machine. Open terminal in the folder where the Package.swift is located and paste the credentials you got fromOption 1: Set AWS environment variables
: It should look something like this
export AWS_ACCESS_KEY_ID="123"
export AWS_SECRET_ACCESS_KEY="abc"
export AWS_SESSION_TOKEN="==sd45f65sd4f56sd4f6sd465fa"
-
Select the
Diva
repository, filter byFormat:swift
, and selectView connection instructions
-
Select
Operating system
,configuration method
and copy the login command in Step 3
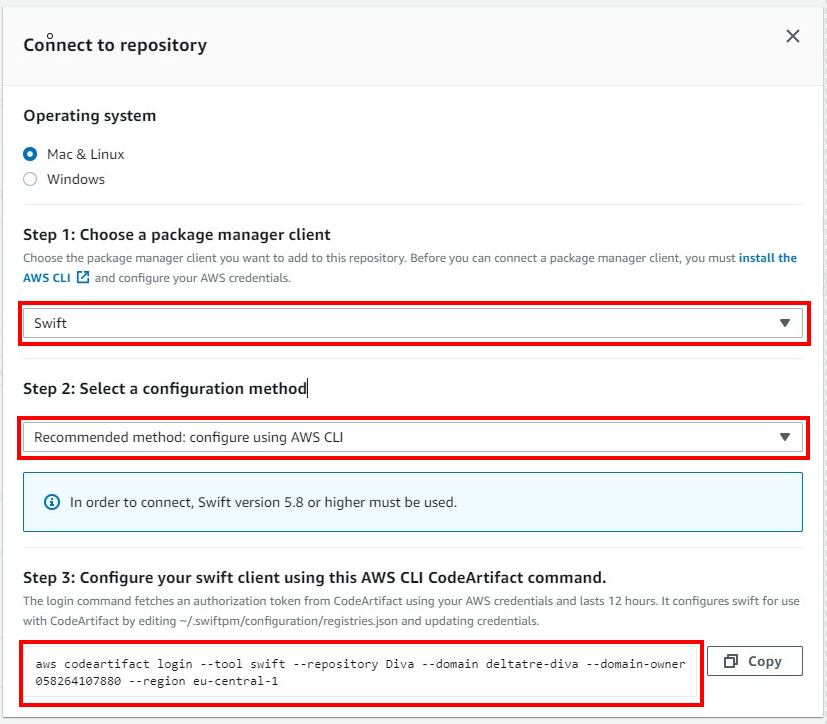
Note: The login session expires within 12 hours.
aws codeartifact login --tool swift --repository Diva --domain deltatre-diva --domain-owner 058264107880 --region eu-central-1
This command will configure your local environment with the necessary settings for Swift to interact with your CodeArtifact repository, including setting the CODEARTIFACT_AUTH_TOKEN
environment variable temporarily.
Additional documentation
Step 2: Write your very first iOS/tvOS app with DIVA Player​
What you need before starting​
- Ensure the DIVA Back Office instance you rely on is up and running.
- Ask your video engineers team the
videoId
andsettings
.
Instantiation​
Replace videoId
and settings
with values you got from your video engineers team in the following code:
class ExampleVideoMetadataProvider {
}
extension ExampleVideoMetadataProvider: VideoMetadataProvider {
func requestVideoMetadata(
videoId: String,
currentVideoMetadata: VideoMetadata?,
playbackState: PlaybackState
) async throws -> VideoMetadata {
SWVideoMetadataCleanModel.make(
videoId: videoId,
title: "title",
image: "https://www.image.jpg",
eventId: "eventId",
programDateTime: "2018-07-15T13:40:15.681Z",
trimIn: 0,
trimOut: 100,
assetState: .vod,
ad: "https://divademo.deltatre.net/DIVAProduct/www/Data/Vast/skippable2.xml",
sources: [
SWVideoSourceCleanModel.make(
uri: "https://vod-ffwddevamsmediaservice.streaming.mediaservices.windows.net/6e8b64ef-feb3-4ea9-9c63-32ef7b45e1dd/6e8b64ef-feb3-4ea9-9c63-32ef7b45.ism/manifest(format=m3u8-aapl,filter=hls)",
format: "HLS"
)
],
audioTracks: [SWAudioTrackCleanModel(_id: "English1" , selector: "English", label: "English")],
defaultAudioTrackId: "English1",
dvrType: .full
)
}
}
class FullscreenViewController: UIViewController {
var diva: Diva?
override func viewDidLoad() {
super.viewDidLoad()
Diva.isDebugMode = false
Diva.mediaAnalyticsEnabled = true
var config = DivaConfiguration(
settings: settings,
dictionary: dictionary,
videoId: videoId,
videoMetadataProvider: ExampleVideoMetadataProvider()
)
config.settingsError = "Settings Error"
config.networkError = "Network Error"
config.onVideoError = { error, videoMetadata in
print("[VideoError] VideoError received for video: \(videoMetadata?.videoId ?? "unknown Id")")
print("[VideoError] error: \(error.type.rawValue) message: \(error.message)")
}
config.onAnalyticEvent = { [weak self] event in
guard self != nil else { return }
var parameters = event.eventArguments
parameters["interactive"] = "\(event.interactive)"
Analytics.logEvent(event.type, parameters: parameters)
print("[EVENT] \(event.type)")
}
config.onMediaAnalyticsUpdate = { [weak self] update in
guard self != nil else { return }
await ConvivaAnalytics.shared.handle(update)
await YouboraAnalytics.shared.handle(update)
}
config.onExit = { [weak self] in
self?.dismiss(animated: true)
}
Task { @MainActor [weak self] in
guard let self else { return }
self.diva = await Diva(configuration: config)
self.diva?.setTargetView(view: self.view, viewController: self)
}
}
deinit {
diva = nil
}
}