Getting started
Step 1: Setup the development environment​
What you need before starting​
- Your AWS account is SSO-enabled to access https://deltatre.awsapps.com/start. After being SSO-enabled (please refer to your Project Manager), restart your development machine.
Setup​
- Access https://deltatre.awsapps.com/start and select
Access keys
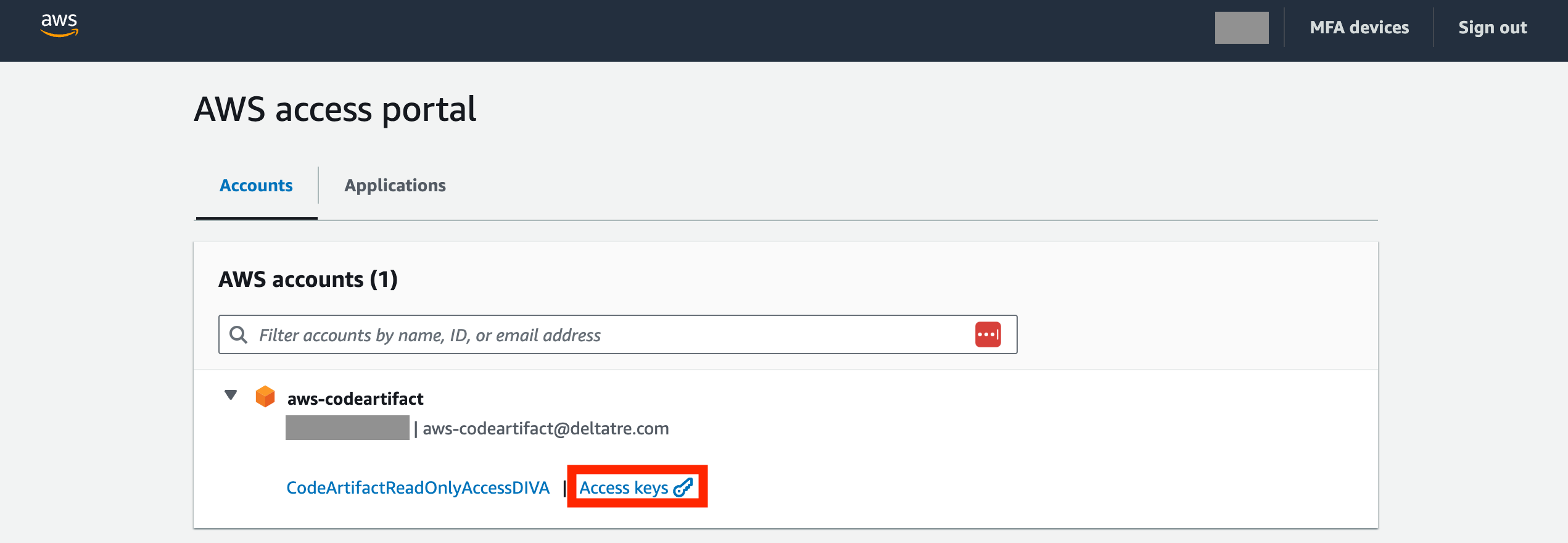
- In a terminal, execute the three imports in
Option 1: Set AWS environment variables
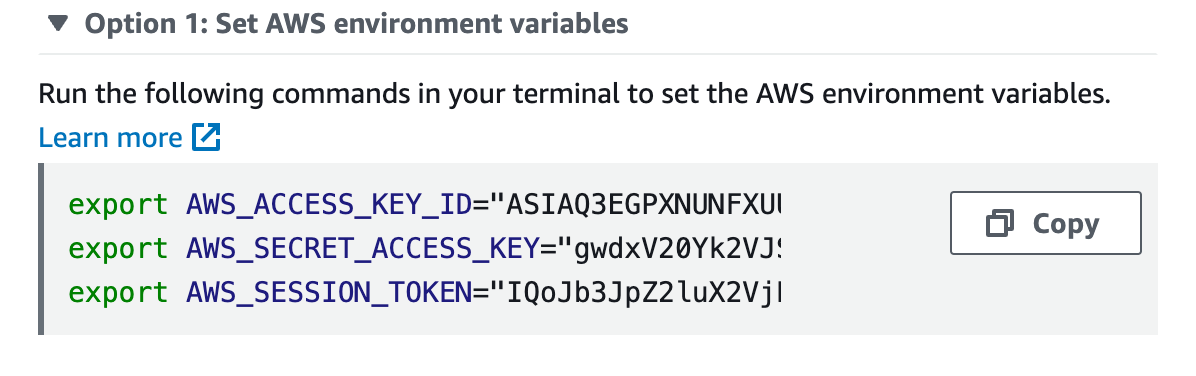
- Go back to https://deltatre.awsapps.com/start and select
CodeArtifactReadOnlyAccessDIVA
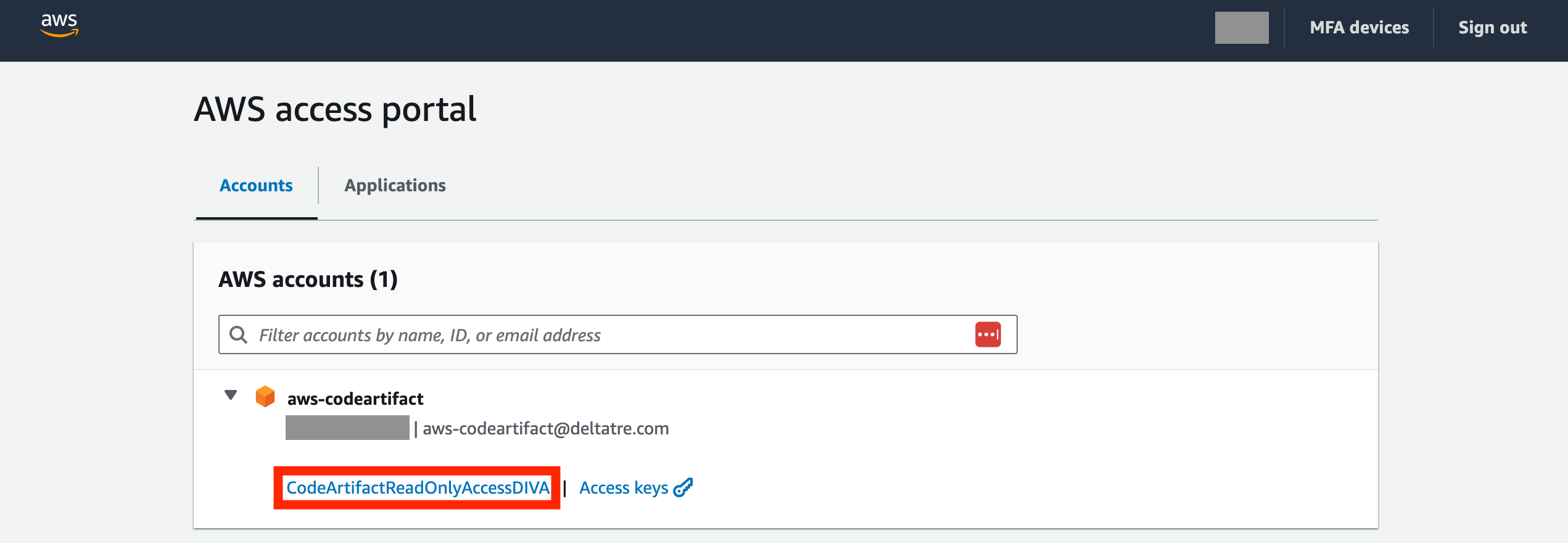
- Search and select
CodeArtifact
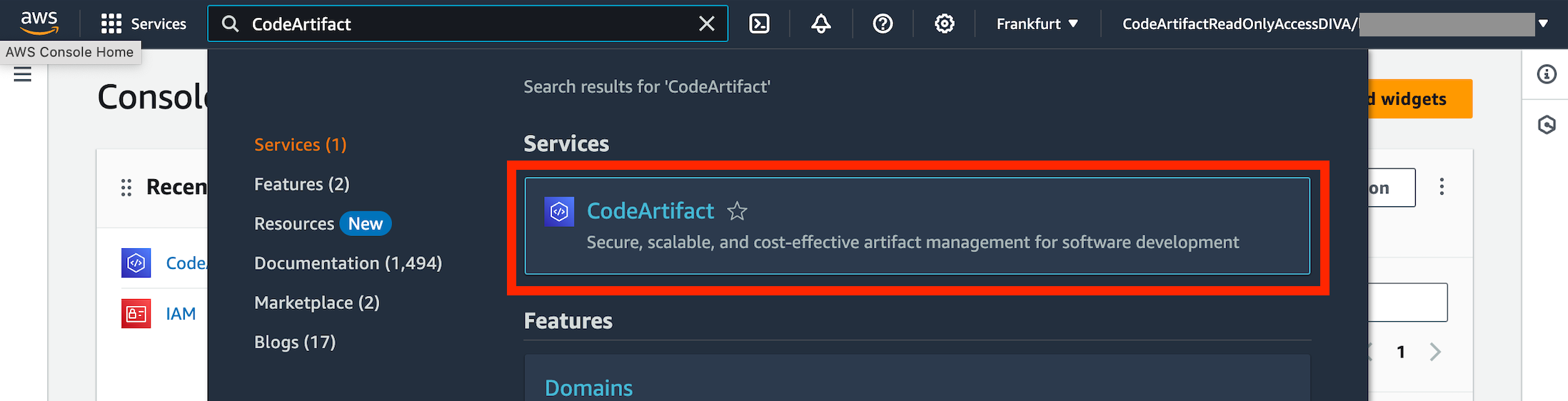
- Select the
Diva
repository, filter byFormat:npm
, and selectView connection instructions
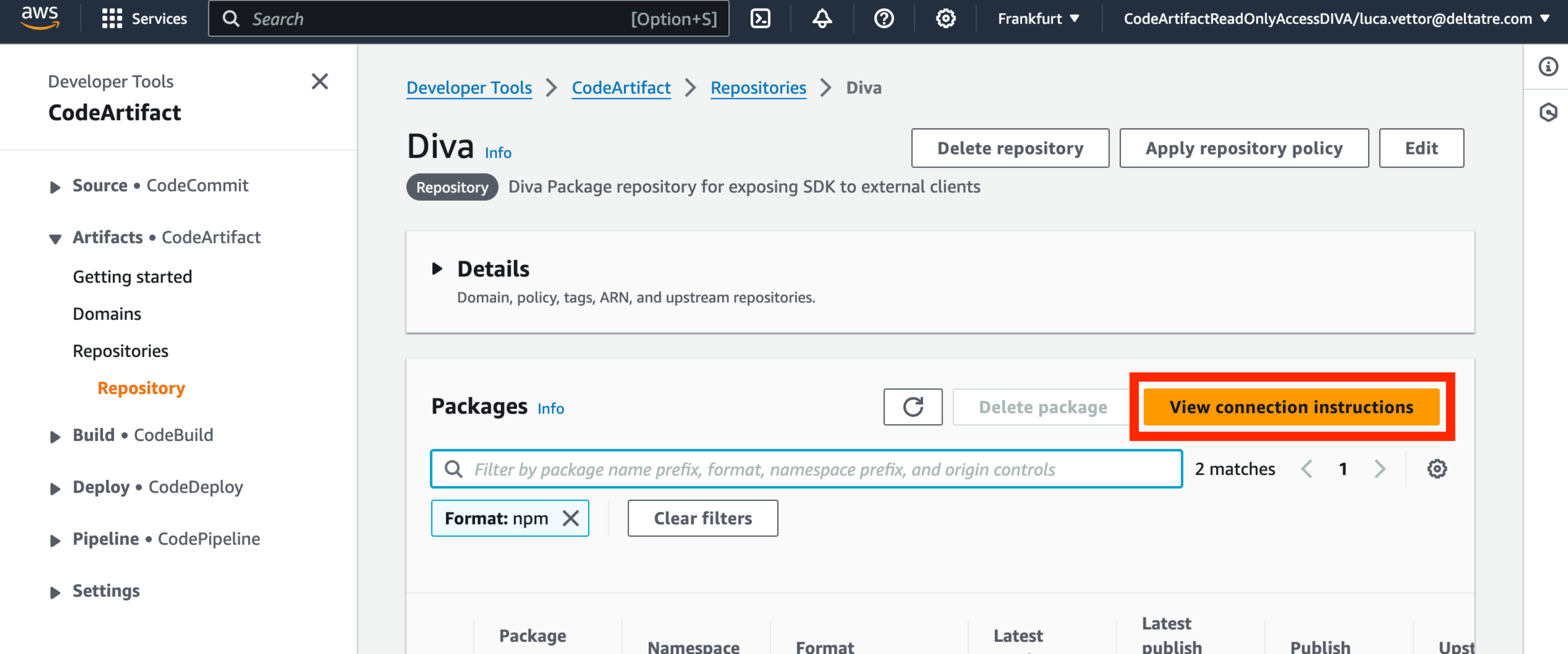
- Select
Operating system
,npm
as the package manager,Recommended method: configure using AWS CLI
, and copy the login command in Step 3
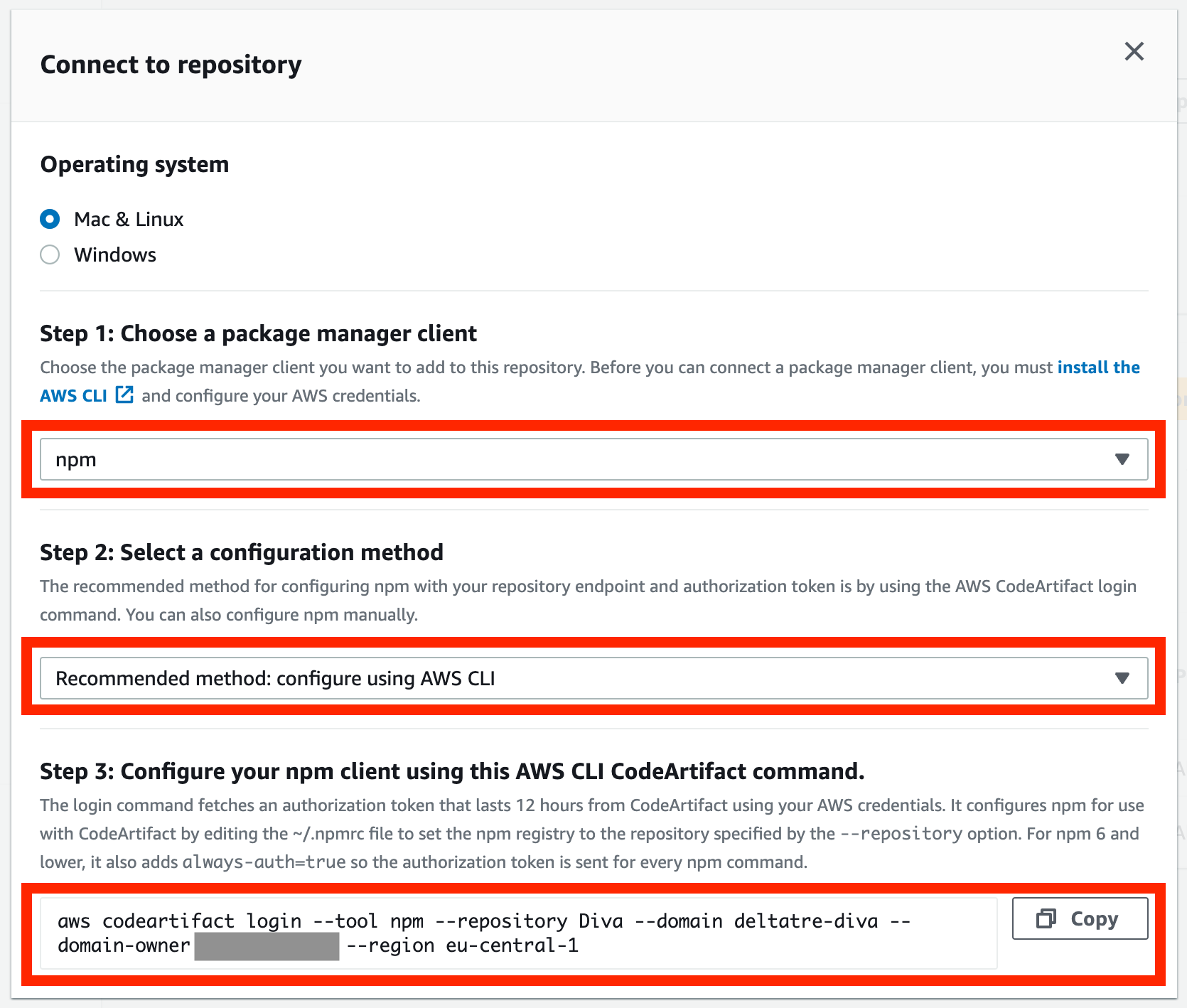
-
Before executing the login command, append
--namespace @deltatre-vxp
at the end, likeaws codeartifact login --tool npm --repository Diva --domain deltatre-diva --domain-owner <YOUR USER CODE> --region eu-central-1 --namespace @deltatre-vxp
Note: The login session expires within 12 hours. Repeat the steps until the login command every time you need to update the DIVA package.
-
Select your project folder and run command
npm i @deltatre-vxp/diva-sdk
-
You're ready to get started coding the front-end with the DIVA Player SDK.
Notes​
In your front-end js/typescript app, make sure to remove the tag <React.StrictMode>
from the root.render
function in the index.tsx
file. Otherwise, the video player's sound may be out of control.
Step 2: Write your very first web app with DIVA Player​
What you need before starting​
- Ensure the Custom Video Production environment you rely on is up and running.
- Set the videoId and
<stream URL>
.
Learn how to configure the DIVA player in the Diva Configuration section.
Instantiation​
Replace <video id>
and <stream URL>
with values you got from your video engineers team in the following code:
Web​
- React App
- No React
- No package manager
// DIVA Player SDK
import { DivaWebComponent } from "@deltatre-vxp/diva-sdk/diva-web-sdk";
import type { DivaConfiguration, VideoMetadata, VideoMetadataClean } from "@deltatre-vxp/diva-sdk/diva-web-sdk";
// Import SDK style
import "@deltatre-vxp/diva-sdk/diva-web-sdk/index.min.css";
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
googleCast: 'https://www.gstatic.com/cv/js/sender/v1/cast_sender.js?loadCastFramework=1',
threeJs: 'https://cdnjs.cloudflare.com/ajax/libs/three.js/0.148.0/three.min.js',
};
const config: DivaConfiguration = {
videoId: "<video id>",
libs: libs,
setting: {
general: {
culture: 'en-GB',
}
},
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId: string,
currentVideoMetadata?: VideoMetadataClean,
playbackState?: {
chromecast?: boolean | undefined;
}): Promise<VideoMetadata> => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
export const App = () => {
return <DivaWebComponent config={config} />;
};
// DIVA Player SDK
import { DivaWeb } from "@deltatre-vxp/diva-sdk/diva-web-sdk";
import type { DivaConfiguration, VideoMetadata, VideoMetadataClean } from "@deltatre-vxp/diva-sdk/diva-web-sdk";
// Import SDK style
import "@deltatre-vxp/diva-sdk/diva-web-sdk/index.min.css";
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
googleCast: 'https://www.gstatic.com/cv/js/sender/v1/cast_sender.js?loadCastFramework=1',
threeJs: 'https://cdnjs.cloudflare.com/ajax/libs/three.js/0.148.0/three.min.js',
};
const config: DivaConfiguration = {
videoId: "<video id>",
libs: libs,
setting: {
general: {
culture: 'en-GB',
}
},
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId: string,
currentVideoMetadata?: VideoMetadataClean,
playbackState?: {
chromecast?: boolean | undefined;
}): Promise<VideoMetadata> => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
const el = document.getElementById("video-player-container");
(async () => {
try {
const divaPlayer = new DivaWeb(el, config);
// To be run when it is required to cleanup Diva after its usage
const onUnmount = () => {
divaPlayer.unmount();
}
} catch (e) {
// Here it is possible to react to DIVA Player exceptions
}
})();
<html>
<head>
<!-- DIVA Player SDK -->
<script src="path/to/diva/webPlayer.reactBundled.js"></script>
<!-- Import SDK style -->
<link rel="path/to/diva.bundle.min.css" />
</head>
<body>
<!-- ... -->
<div id="diva-container"></div>
<!-- ... -->
<script>
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
googleCast: 'https://www.gstatic.com/cv/js/sender/v1/cast_sender.js?loadCastFramework=1',
threeJs: 'https://cdnjs.cloudflare.com/ajax/libs/three.js/0.148.0/three.min.js',
};
const config = {
videoId: "<video id>",
libs: libs,
setting: {
general: {
culture: 'en-GB',
}
},
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId,
currentVideoMetadata,
playbackState) => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
const el = document.getElementById("video-player-container");
(async () => {
try {
const divaPlayer = await new divaWebSdk.DivaWeb(el, config);
// To be run when it is required to cleanup Diva after its usage
const onUnmount = () => {
divaPlayer.unmount();
}
} catch (e) {
// Here it is possible to react to DIVA Player exceptions
}
})();
</script>
</body>
</html>
Web Chromeless​
- React App
- No React
- No package manager
// DIVA Player SDK
import { DivaWebChromelessComponent } from "@deltatre-vxp/diva-sdk/diva-web-chromeless-sdk";
import type { DivaWebChromelessConfiguration, VideoMetadata, VideoMetadataClean } from "@deltatre-vxp/diva-sdk/diva-web-chromeless-sdk";
const isWebTV = false;
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
googleCast: 'https://www.gstatic.com/cv/js/sender/v1/cast_sender.js?loadCastFramework=1',
threeJs: 'https://cdnjs.cloudflare.com/ajax/libs/three.js/0.148.0/three.min.js',
};
const config: DivaConfiguration = {
videoId: "<video id>",
libs: libs,
setting: {
general: {
culture: 'en-GB',
}
},
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId: string,
currentVideoMetadata?: VideoMetadataClean,
playbackState?: {
chromecast?: boolean | undefined;
}): Promise<VideoMetadata> => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
const props = {
config: config,
muted=false,
volume=1,
adCover: true,
videoCover: true,
}
export const App = () => {
return <DivaWebChromelessComponent { ...props } />;
};
// DIVA Player SDK
import { createDivaWebChromeless } from "@deltatre-vxp/diva-sdk/diva-web-chromeless-sdk";
const isWebTV = false;
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
googleCast: 'https://www.gstatic.com/cv/js/sender/v1/cast_sender.js?loadCastFramework=1',
threeJs: 'https://cdnjs.cloudflare.com/ajax/libs/three.js/0.148.0/three.min.js',
};
const config: DivaConfiguration = {
videoId: "<video id>",
libs: libs,
setting: {
general: {
culture: 'en-GB',
}
},
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId: string,
currentVideoMetadata?: VideoMetadataClean,
playbackState?: {
chromecast?: boolean | undefined;
}): Promise<VideoMetadata> => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
const el = document.getElementById("video-player-container");
const props = {
el: el,
config: config,
}
(async () => {
try {
const diva = await createDivaWebChromeless(props);
diva.setStyle({ adCover: true, videoCover: true });
diva.setVolume(0.5);
// To be run when it is required to cleanup Diva after its usage
const onUnmount = () => {
diva.stop();
}
} catch (e) {
// Here it is possible to react to DIVA Player exceptions
}
})();
<html>
<head>
<!-- ... -->
<script src="path/to/diva/chromeless.reactBundled.js"></script>
</head>
<body>
<!-- ... -->
<div id="diva-container"></div>
<!-- ... -->
<script>
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
googleCast: 'https://www.gstatic.com/cv/js/sender/v1/cast_sender.js?loadCastFramework=1',
threeJs: 'https://cdnjs.cloudflare.com/ajax/libs/three.js/0.148.0/three.min.js',
};
const config = {
videoId: "<video id>",
libs: libs,
setting: {
general: {
culture: 'en-GB',
}
},
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId,
currentVideoMetadata,
playbackState) => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
const el = document.getElementById("video-player-container");
const props = {
el: el,
config: config
}
(async () => {
try {
const diva = await divaWebChromelessSdk.createDivaWebChromeless(props);
diva.setStyle({ adCover: true, videoCover: true });
diva.setVolume(0.5);
// To be run when it is required to cleanup Diva after its usage
const onUnmount = () => {
diva.stop();
}
} catch (e) {
// Here it is possible to react to DIVA Player exceptions
}
})();
</script>
</body>
</html>
WebTV​
- React App
- No React
- No package manager
// DIVA Standalone SDK
import { DivaWebTV } from "@deltatre-vxp/diva-sdk/diva-webtv-sdk";
import type {
DivaWebTVInitType,
DivaWebTVProps,
VideoMetadata,
VideoMetadataClean
} from "@deltatre-vxp/diva-sdk/diva-webtv-sdk";
// Import SDK style
import "@deltatre-vxp/diva-sdk/diva-webtv-sdk/index.min.css";
const init: DivaWebTVInitType = {
setting: {
general: {
culture: "en-GB",
}
},
videoId: "<video id>"
};
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
};
const props: DivaWebTVProps = {
init,
libs,
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId: string,
currentVideoMetadata?: VideoMetadataClean,
playbackState?: {
chromecast?: boolean | undefined;
}): Promise<VideoMetadata> => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
export const App = () => {
return <DivaWebTV {...props} />;
}
// DIVA Player SDK
import { createDivaWebTV } from "@deltatre-vxp/diva-sdk/diva-webtv-sdk";
import type {
DivaWebTVInitType,
DivaWebTVProps,
VideoMetadata,
VideoMetadataClean
} from "@deltatre-vxp/diva-sdk/diva-webtv-sdk";
// Import SDK style
import "@deltatre-vxp/diva-sdk/diva-webtv-sdk/index.min.css";
const init: DivaWebTVInitType = {
setting: {
general: {
culture: "en-GB",
}
},
videoId: "<video id>"
};
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
};
const props: DivaWebTVProps = {
init,
libs,
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId: string,
currentVideoMetadata?: VideoMetadataClean,
playbackState?: {
chromecast?: boolean | undefined;
}): Promise<VideoMetadata> => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
(async () => {
try {
const diva = await createDivaWebTV(props);
// To be run when it is required to cleanup Diva after its usage
const onUnmount = () => {
diva.unmount();
}
} catch (e) {
// Here it is possible to react to DIVA Player exceptions
}
})();
<html>
<head>
<!-- ... -->
<script src="path/to/diva/webTVSdk.reactBundled.js"></script>
<link rel="path/to/diva/webTVSdk.reactBundled.css" />
</head>
<body>
<!-- ... -->
<div id="diva-container"></div>
<!-- ... -->
<script>
const init = {
setting: {
general: {
culture: "en-GB",
}
},
videoId: "<video id>"
};
const libs = {
mux: "https://cdnjs.cloudflare.com/ajax/libs/mux.js/6.2.0/mux.min.js",
shaka: "https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.11.2/shaka-player.compiled.js",
hlsJs: "https://cdn.jsdelivr.net/npm/hls.js@1.5.7",
googleIMA: 'https://imasdk.googleapis.com/js/sdkloader/ima3.js',
googleDAI: 'https://imasdk.googleapis.com/js/sdkloader/ima3_dai.js',
};
const props = {
init,
libs,
dictionary: {
messages: {},
},
videoMetadataProvider: async (
videoId,
currentVideoMetadata,
playbackState): Promise<VideoMetadata> => {
return {
"title": "Video Title",
"sources": [
{
"uri": "https://bitdash-a.akamaihd.net/content/MI201109210084_1/mpds/f08e80da-bf1d-4e3d-8899-f0f6155f6efa.mpd",
"format": "DASH"
}
],
"videoId": "<video id>",
};
}
};
const el = document.getElementById("video-player-container");
(async () => {
try {
const diva = await divaWebtv.createDivaWebTV(props);
// To be run when it is required to cleanup Diva after its usage
const onUnmount = () => {
diva.unmount();
}
} catch (e) {
// Here it is possible to react to DIVA Player exceptions
}
})();
</script>
</body>
</html>